For client-side only, does not work in Node.js
In front-end development, validating colors provided by users or external inputs can help maintain consistency in the UI. This utility function uses the browser’s native capabilities to determine whether a string represents a valid color in CSS.
Basic Color Validator Function
This function checks if a string is a valid CSS color by creating a temporary DOM element and applying the color to it.
let isValidColor = (color) => {
let otpNode = new Option() // Create a temporary option element
otpNode.style.color = color // Set the color style
return !!otpNode.style.color // Return true if the color is valid
}
- Explanation: We create a
<option>
HTML element dynamically and assign the input string as the color. The browser will only apply valid CSS colors, so by checking if thestyle.color
property is set, we can determine if the color is valid.
Example Usages
You can test this function with a variety of color formats:
// Valid colors
isValidColor('purple') // true
isValidColor('burlywood') // true
isValidColor('lightsalmon') // true
isValidColor('rgb(255, 255, 255)') // true
isValidColor('rgba(255, 255, 255, .5)') // true
isValidColor('#ccc') // true
isValidColor('hsl(100, 0%, 18%)') // true
// Invalid colors
isValidColor('not-a-color-name') // false
isValidColor('dark gray') // false (should be 'darkgray')
isValidColor('rgb(255, 255, 255, 1, 1)') // false
isValidColor('#ccczzz') // false
Caveat
Certain special values like unset
, initial
, inherit
, currentcolor
, and transparent
are considered valid CSS values for color
but may not be desirable in some contexts.
To exclude these values, we can modify the function:
let isValidColor = (color) => {
let otpNode = new Option() // Create a temporary option element
otpNode.style.color = color // Set the color style
return !!otpNode.style.color && !/(unset|initial|inherit|currentcolor|transparent)/i.test(color)
}
Visual Representation of the Validator
Here's a breakdown of how the validator works for common color formats:
Color String | Valid? | Example Code |
---|---|---|
'purple' | ✅ Yes | isValidColor('purple') |
'rgb(255, 255, 255)' | ✅ Yes | isValidColor('rgb(255, 255, 255)') |
'#ccc' | ✅ Yes | isValidColor('#ccc') |
'not-a-color-name' | ❌ No | isValidColor('not-a-color-name') |
'unset' | ✅ Yes | isValidColor('unset') (special value) |
'rgb(-1, 255, 255)' | ✅ Yes | isValidColor('rgb(-1, 255, 255)') (valid but strange) |
'dark gray' | ❌ No | isValidColor('dark gray') (incorrect spelling) |
'transparent' | ✅ Yes | isValidColor('transparent') (valid but special) |
Limitations
- Client-Side Only: This method relies on the DOM, so it will not work in environments like Node.js.
- False Positives: Some invalid color formats, such as
rgb(-1, 255, 255)
may pass as valid since CSS treats them as valid. - Special CSS Values: Special values like
unset
,initial
, andinherit
are valid in CSS but might not be desired as color inputs.
Practical Applications
- User Input Validation: Ensuring users only input valid colors for customizing the appearance of a site or application.
- Form Inputs: Validating color input fields to ensure the provided data will render correctly in the UI.
- Dynamic Theming: Safeguarding against invalid theme colors when users customize the look and feel of an app.
Final Thoughts
This validator function provides a lightweight, client-side solution to check for valid CSS colors. While it works well for many use cases, be mindful of potential edge cases like special CSS keywords or color formats that are technically valid but undesirable.
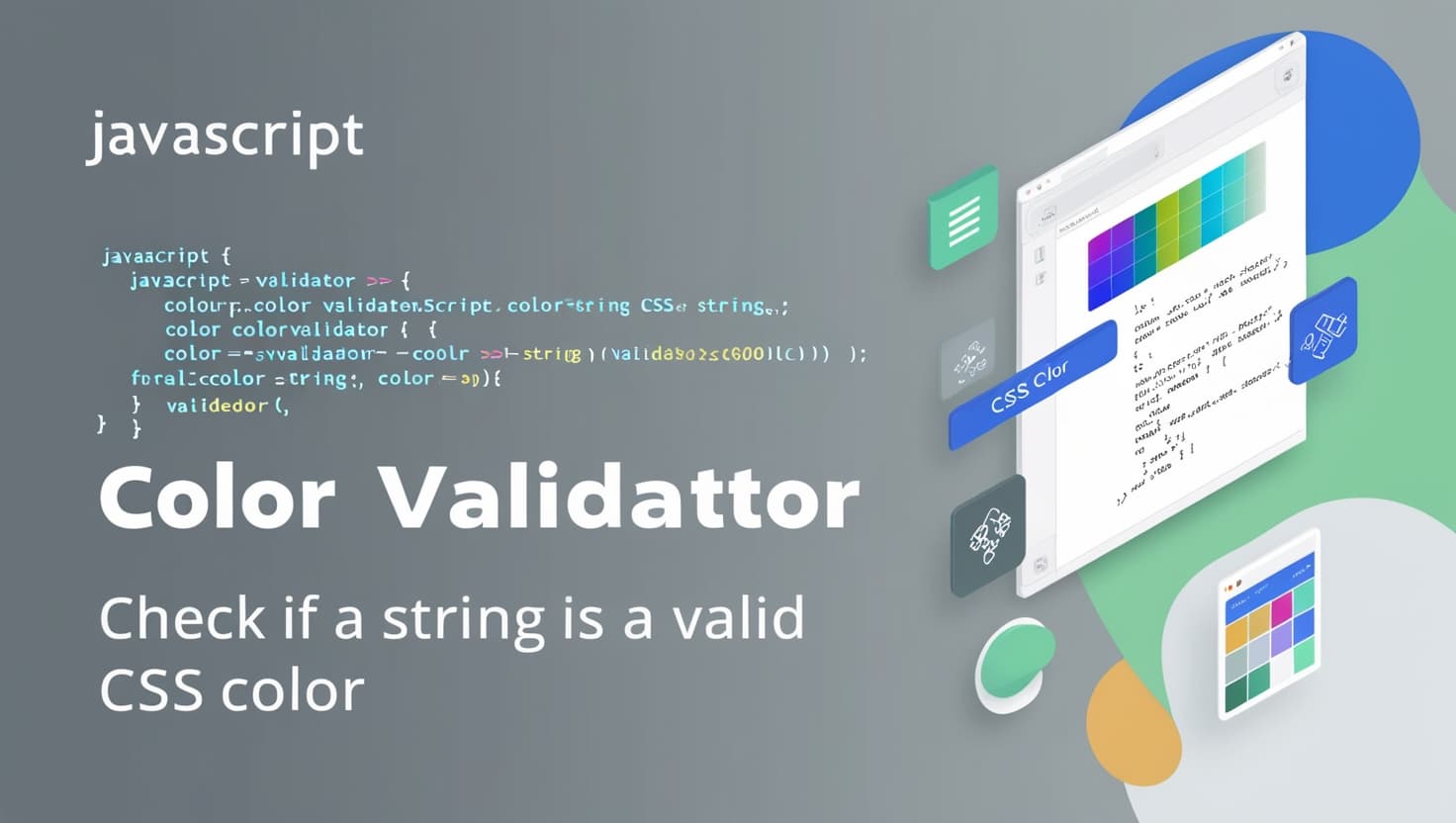
Feel free to expand this function based on your project’s needs or add further checks for more robust validation.