Remove all falsy values from an object and its nested children
- Published on
- • 6 mins read
Removing Falsy Values Deeply in JavaScript and TypeScript
Sometimes objects contain unwanted values that are considered falsy in JavaScript, such as null
, undefined
, 0
, ''
, and false
. Removing these values can clean up data, especially when dealing with deeply nested structures. This utility function helps you do exactly that.
You can also customize which falsy values to remove by passing an array of specific falsy values to the function.
If you prefer typescript
, here's the TypeScript version:
export function removeFalsy<T = any>(obj: {}, falsyValues: any[] = ['', null, undefined]): T {
if (!obj || typeof obj !== 'object') {
return obj as any
}
return Object.entries(obj).reduce((a, c) => {
let [k, v]: [string, any] = c
if (falsyValues.indexOf(v) === -1 && JSON.stringify(removeFalsy(v, falsyValues)) !== '{}') {
a[k] = typeof v === 'object' && !Array.isArray(v) ? removeFalsy(v, falsyValues) : v
}
return a
}, {} as any) as T
}
JavaScript Version
Here’s a JavaScript version of the function that doesn’t rely on as
and any
:
function removeFalsy(obj, falsyValues = ['', null, undefined]) {
if (!obj || typeof obj !== 'object') {
return obj
}
return Object.entries(obj).reduce((a, c) => {
let [k, v] = c
if (falsyValues.indexOf(v) === -1 && JSON.stringify(removeFalsy(v, falsyValues)) !== '{}') {
a[k] = typeof v === 'object' && !Array.isArray(v) ? removeFalsy(v, falsyValues) : v
}
return a
}, {})
}
Example Usage
Here’s how you can use this function to remove falsy values from an object with nested children:
let obj = {
a: 1,
b: 0,
c: '',
d: null,
e: undefined,
f: false,
g: {
a: 1,
b: 0,
c: '',
d: null,
e: undefined,
f: false,
},
j: {},
h: [],
i: [1],
}
console.log(removeFalsy(obj, [0, false, '', null, undefined]))
// 👉 { a: 1, g: { a: 1 }, i: [ 1 ] }
Understanding How It Works
The function works recursively. It checks each property of the object to determine whether it contains one of the specified falsy values. If it doesn’t, the function keeps the value; if it does, the property is removed from the object.
- Customization: You can define what you consider falsy values by passing an array of values (e.g.,
['', 0, null, undefined]
). This makes the function flexible for different use cases. - Deep Removal: The function is recursive, meaning it will look into nested objects and remove falsy values from them as well.
Practical Applications
This function is particularly useful when:
- Cleaning API Responses: You might receive data with a lot of unnecessary null, empty, or undefined values that you don’t want to pass on.
- Optimizing Form Data: When submitting form data, you may want to remove empty or default values before sending it to your backend.
- Reducing Object Size: For performance optimization, especially in cases where data is passed between different systems or stored in databases, removing irrelevant fields can save storage and processing time.
Caveats and Edge Cases
- Empty Arrays and Objects: In its current form, empty arrays (
[]
) and objects ({}
) are not considered falsy, but you can adjust the function logic to remove them if needed. - Circular References: Be cautious if your object contains circular references. This function doesn’t handle them out of the box and may cause infinite recursion.
Happy coding, and remember, keeping your objects clean helps you write better and more maintainable code!
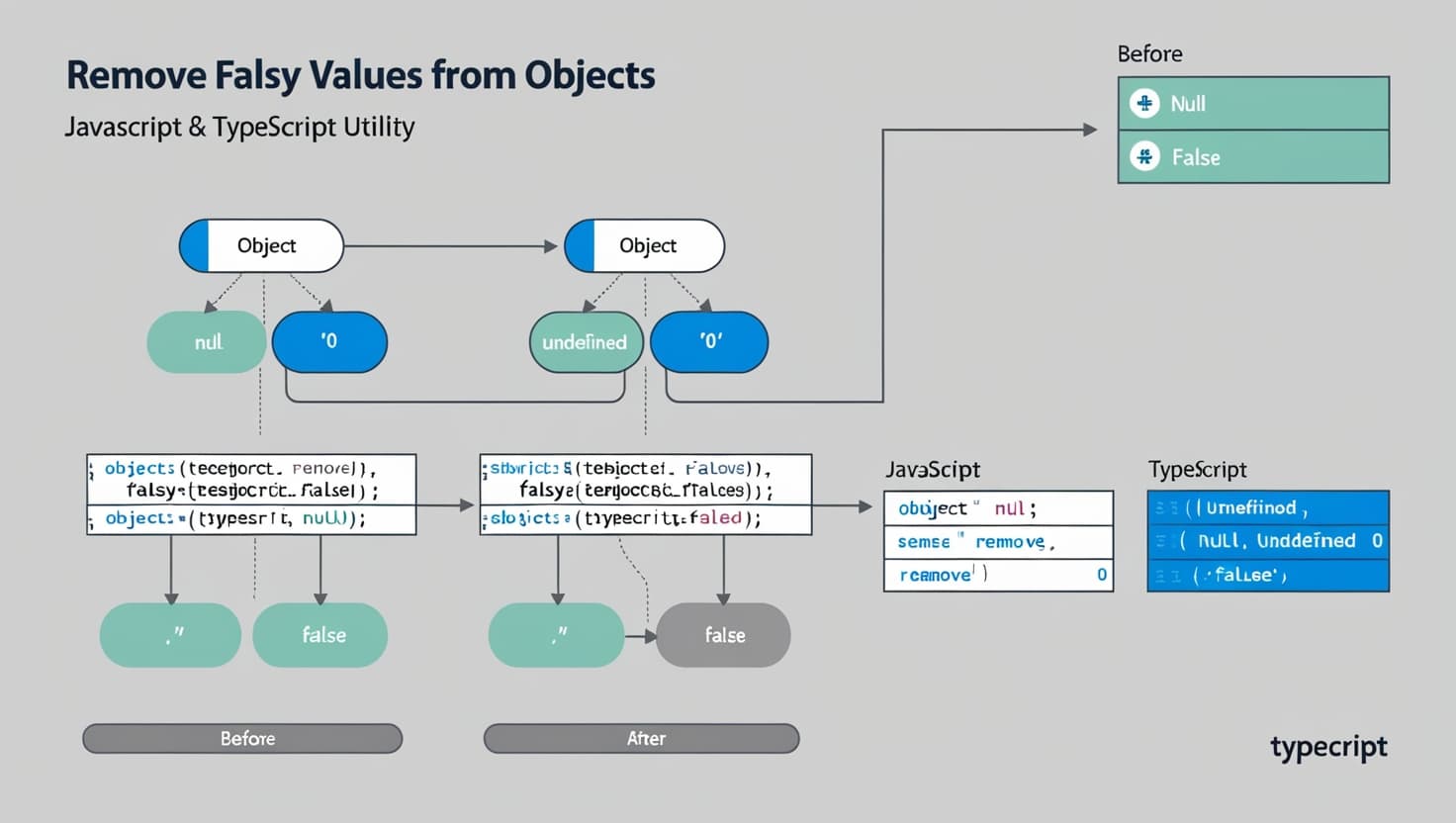
Learn how to create and use a custom hook to handle asynchronous side effects in React: Use an async effect