Reusable JSX Code Snippets
These snippets provide common solutions and patterns used in JSX and React development. From conditional rendering to dynamic styling, these patterns are highly reusable and will simplify many of your UI tasks.
1. Conditional Component Rendering
This pattern is useful when you need to render different components based on a condition.
function ConditionalComponent({ condition }) {
return condition ? <ComponentA /> : <ComponentB />;
}
- Use Case: Toggle between components based on a boolean condition, such as displaying a loader or the actual content once data has loaded.
2. Map Over an Array to Render a List of Components
Rendering lists is common in React applications. The map()
function allows you to iterate over an array and display a list of components.
function ListComponent({ items }) {
return (
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
);
}
- Use Case: Perfect for rendering dynamic content like product listings, blog posts, or any other data-driven list.
3. Render a Component with Inline Styles
Sometimes you may want to add styles directly within your JSX code, using the style
attribute.
function InlineStyleComponent() {
const styles = {
color: 'red',
fontSize: '16px',
};
return <div style={styles}>Hello, World!</div>;
}
- Use Case: Ideal for quick styling solutions or dynamic styling based on JavaScript logic.
4. Conditional Styling Based on Props
This snippet helps you apply different styles depending on a prop, like changing color based on an isActive
state.
function ConditionalStyleComponent({ isActive }) {
const styles = {
color: isActive ? 'blue' : 'gray',
fontSize: '16px',
};
return <div style={styles}>Hello, World!</div>;
}
- Use Case: Useful for toggling visual states (e.g., a button or link changing color based on whether it’s active).
5. Stateful Component with Event Handler
This snippet shows how to use the useState
hook to manage component state and handle user interactions like click events.
import { useState } from 'react';
function StatefulComponent() {
const [count, setCount] = useState(0);
const handleClick = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={handleClick}>Increment</button>
</div>
);
}
- Use Case: Commonly used for interactive components like counters, forms, or any component that needs state management.
6. Dynamic Class Names Based on Props
This is helpful when you need to apply different class names to a component based on its state or props.
function DynamicClassComponent({ isActive }) {
const classNames = `component ${isActive ? 'active' : 'inactive'}`;
return <div className={classNames}>Hello, World!</div>;
}
- Use Case: Useful for toggling CSS classes in response to state changes, like toggling a sidebar open or closed.
Conclusion
These reusable JSX patterns are great tools for React developers. They simplify common tasks such as rendering conditional components, handling user events, or managing dynamic styles. By mastering these snippets, you can enhance your productivity and make your code more maintainable.
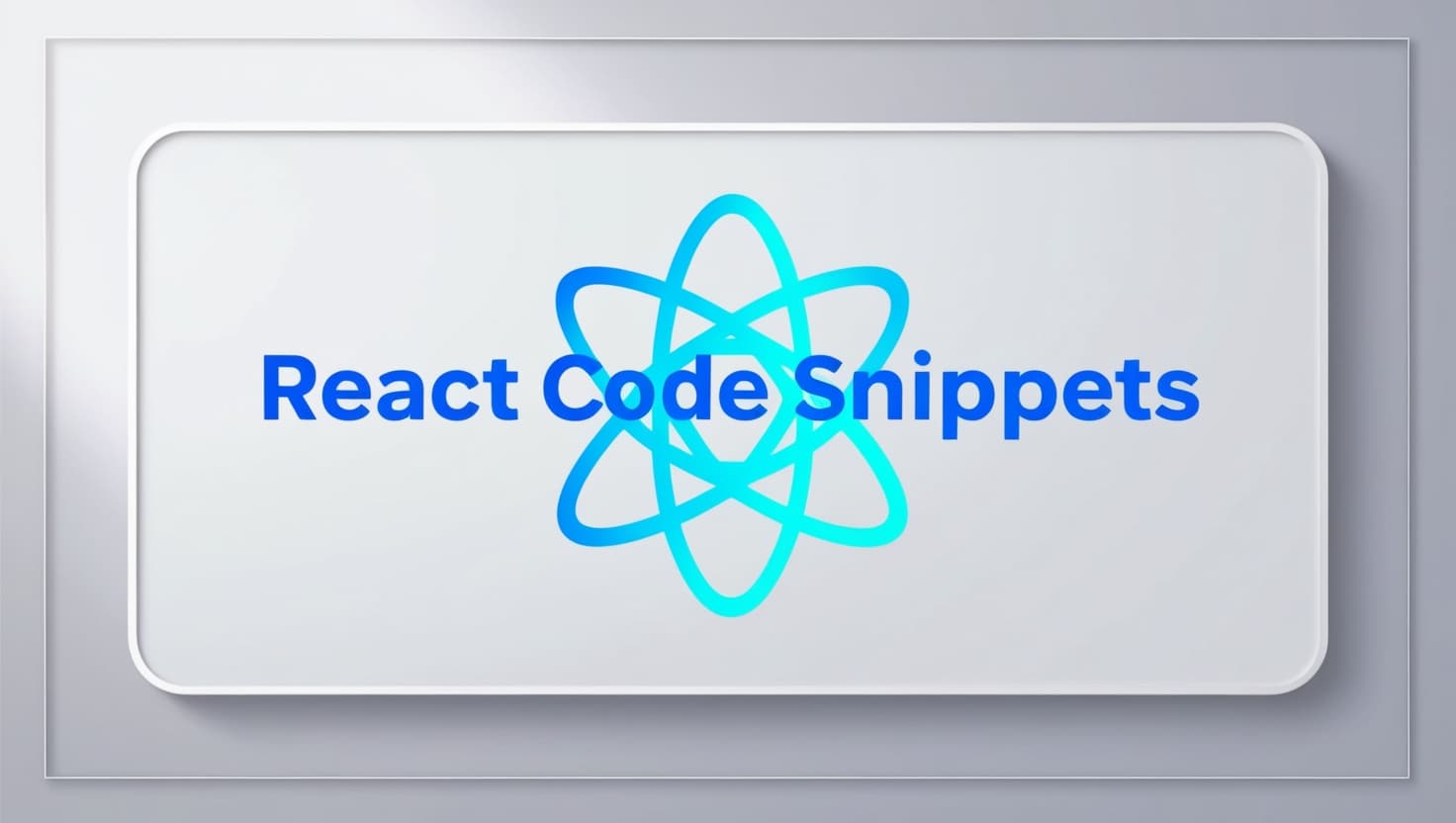
For more React snippets and reusable patterns, stay tuned!
How to get Spotify token and use it to display now playing track on your website: Getting Spotify token